Building and Integrating a Binary Rain Loader in Angular
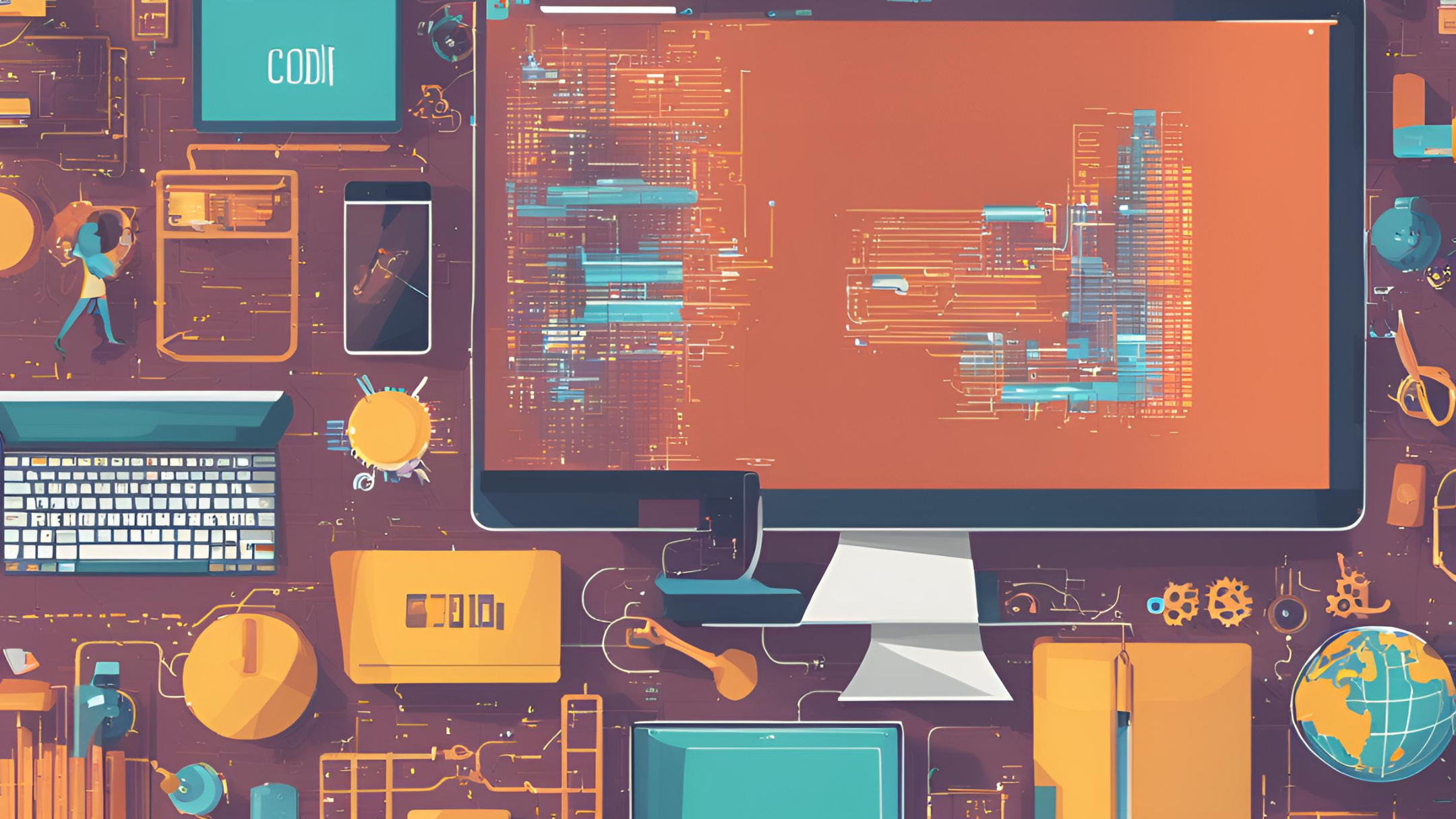
Loading indicators are an essential part of modern web applications. They keep users engaged while data is being processed or fetched. In this article, we’ll build a visually appealing binary rain loader and integrate it into an Angular project using a real-world example.
Overview
The binary rain loader is inspired by the visual aesthetic of falling binary code. Each column updates dynamically to create an animated rain effect. Let’s see how to implement and integrate it into your Angular project.
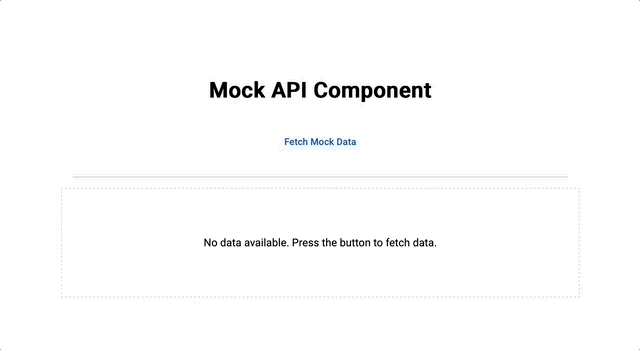
Prerequisites
Before starting, ensure you have the following:
- Node.js and npm installed on your machine.
- Angular CLI installed globally. If not, install it using:
npm install -g @angular/cli
- A basic Angular project set up. If you need a new project, create one using:
ng new binary-rain-loader-demo
cd binary-rain-loader-demo
- Angular Material for styling (optional). Install it by running:
cd src #inside the project folder
ng add @angular/material
Follow the prompts to configure your project. I opted for scss styling.
Step 1: Building the loader component
Let’s create the binary rain loader component.
TypeScript (loader logic)
The loader uses a grid-like structure where each cell randomly updates to show 0
or 1
. Create a _components
folder under /src/app/
and generate the component using Angular CLI inside:
ng g c loader
Update the loader.component.ts
file as follows:
import { NgFor } from '@angular/common';
import { Component, Input, OnInit } from '@angular/core';
@Component({
selector: 'app-loader',
imports: [NgFor],
templateUrl: './loader.component.html',
styleUrl: './loader.component.scss',
})
export class LoaderComponent implements OnInit{
constructor() { }
loaderData: string[][] = [];
ngOnInit(): void {
// Initialize the loader with a random binary array
this.initializeLoader();
// Update each column at different intervals
// smaller is faster
const columnIntervals = [400, 400, 400, 400];
for (let i = 0; i < 4; i++) {
setInterval(() => {
this.updateColumn(i);
}, columnIntervals[i]);
}
}
private initializeLoader() {
for (let i = 0; i < 4; i++) {
const row = [];
for (let j = 0; j < 4; j++) {
row.push(this.getRandomBinary());
}
this.loaderData.push(row);
}
}
private getRandomBinary(): string {
return Math.random() < 0.5 ? '0' : '1';
}
private updateColumn(columnIndex: number) {
// Shift the data in the specified column vertically
const newRow:string[] = [];
for (let i = 0; i < 4; i++) {
newRow.push(this.getRandomBinary());
}
this.loaderData.forEach((row) => {
row[columnIndex] = newRow.shift()!;
});
}
}
HTML (loader structure)
The HTML file creates a flexible grid layout to display the binary rain. Add the following in loader.component.html
:
<div class="binary-rain-loader">
<div *ngFor="let row of loaderData" class="binary-row">
<div *ngFor="let cell of row" class="binary-cell">
{{ cell }}
</div>
</div>
</div>
SCSS (loader animation and styling)
Add animations to bring the loader to life. Update loader.component.scss
:
.binary-rain-loader {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
animation: pulse 2s infinite;
}
.binary-row {
display: flex;
}
.binary-cell {
width: 1ch;
height: 1ch;
padding: 1ch;
display: flex;
align-items: center;
justify-content: center;
font-size: 1em; //by default it is as the container's
text-shadow: 1px 1px 1px rgba(0, 0, 0, 0.5);
}
@keyframes pulse {
0% {
opacity: 1;
}
50% {
opacity: 0.5;
}
100% {
opacity: 1;
}
}
// for combination of pulsing and scaling
// @keyframes pulse {
// 0% {
// transform: scale(1);
// opacity: 1;
// }
// 50% {
// transform: scale(0.8);
// opacity: 0.5;
// }
// 100% {
// transform: scale(1);
// opacity: 1;
// }
// }
You can modify @keyframes
according to your preferences! You can add a pulse effect for example by scaling up and down. You can also experiment with different colors of binary rain by changing color
property of .binary-cell
.
Step 2: Integrating the loader
Now that the loader is ready, let’s integrate it into a real-world scenario where it shows while fetching data. For simplicity's sake, we are going to create a mock API service with random delay in its response and the corresponding consumer component.
Creating the mock API service
Under /src/app/
create a _services
folder. Navigate inside and generate a new service running:
ng g s mock-api
On the newly created mock-api.service.ts
add the following:
import { Injectable } from '@angular/core';
import { Observable, of, delay } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class MockApiService {
constructor() { }
getMockData(): Observable<string> {
const randomDelay = Math.floor(Math.random() * 2500) + 1500;
const text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.";
return of(text).pipe(delay(randomDelay));
}
}
Create the consumer component
Now, let's navigate again under /src/app/_components
folder and create our consumer:
ng g c api-consumer
Update component's logic on api-consumer.component.ts
:
import { Component, OnDestroy } from '@angular/core';
import { LoaderComponent } from '../loader/loader.component';
import { Subject, takeUntil } from 'rxjs';
import { MockApiService } from '../../_services/mock-api.service';
import { MatSnackBar } from '@angular/material/snack-bar';
import { NgIf } from '@angular/common';
import { MatButton } from '@angular/material/button';
@Component({
selector: 'app-api-consumer',
imports: [LoaderComponent, NgIf, MatButton],
templateUrl: './api-consumer.component.html',
styleUrl: './api-consumer.component.scss'
})
export class ApiConsumerComponent implements OnDestroy{
destroy$: Subject<void> = new Subject<void>();
data: string | undefined = undefined;
isLoading: boolean = false;
constructor(private mockApiService: MockApiService, private snackBar: MatSnackBar) { }
fetchData(): void {
this.isLoading = true;
this.data = undefined;
this.mockApiService.getMockData()
.pipe(takeUntil(this.destroy$))
.subscribe({
next: (response: string) => {
this.data = response
this.isLoading = false;
},
error: (error) => {
this.isLoading = false;
this.snackBar.open('There was an error!' + error, 'Close', {
horizontalPosition: 'center',
verticalPosition: 'top',
duration: 3000,
});
}
})
}
ngOnDestroy(): void {
this.destroy$.next();
this.destroy$.complete();
}
}
We take care of possible memory leaks by implementing onDestroy
lifecycle for our observables.
Now we want a simple HTML structure for api-consumer.component.html
to consume the API service and display our mock data :
<div class="container">
<h1>Mock API Component</h1>
<div class="button-container">
<button mat-button (click)="fetchData()">Fetch Mock Data</button>
</div>
<hr />
<div class="placeholder-container" *ngIf="!isLoading; else loading">
<p *ngIf="data">{{ data }}</p>
<p *ngIf="!data">No data available. Press the button to fetch data.</p>
</div>
<ng-template #loading>
<app-loader></app-loader>
</ng-template>
</div>
Finally, let's make it more user-friendly by adding the proper styling under api-consumer.component.scss
:
.container {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
text-align: center;
background-color: #f9f9f9;
padding: 1rem;
}
.button-container {
margin: 1rem;
}
hr {
width: 80%;
margin: 1rem 0;
border: none;
border-top: 1px solid #ccc;
}
.placeholder-container {
width: 80%;
min-height: 128px;
display: flex;
justify-content: center;
align-items: center;
border: 1px dashed #ccc;
padding: 1rem;
background-color: #fff;
}
Running the Project
Now we are ready to run our project locally!
Run the Angular development server:
ng serve
Once the server is running, open your browser and navigate to http://localhost:4200/. The application will automatically reload whenever you modify any of the source files.
Click the "Fetch Mock Data" button and see the loader in action!
GitHub Repository
To explore the full implementation and download the source code, visit my GitHub repository.
Conclusion
The Binary Rain Loader is a visually appealing and reusable loading indicator for Angular projects. By following this guide, you’ve learned how to build it from scratch and integrate it into real-world scenarios.
Start enhancing your Angular projects today with this engaging animation!